How to Serve Django and React on the Same Port
01/15/2021
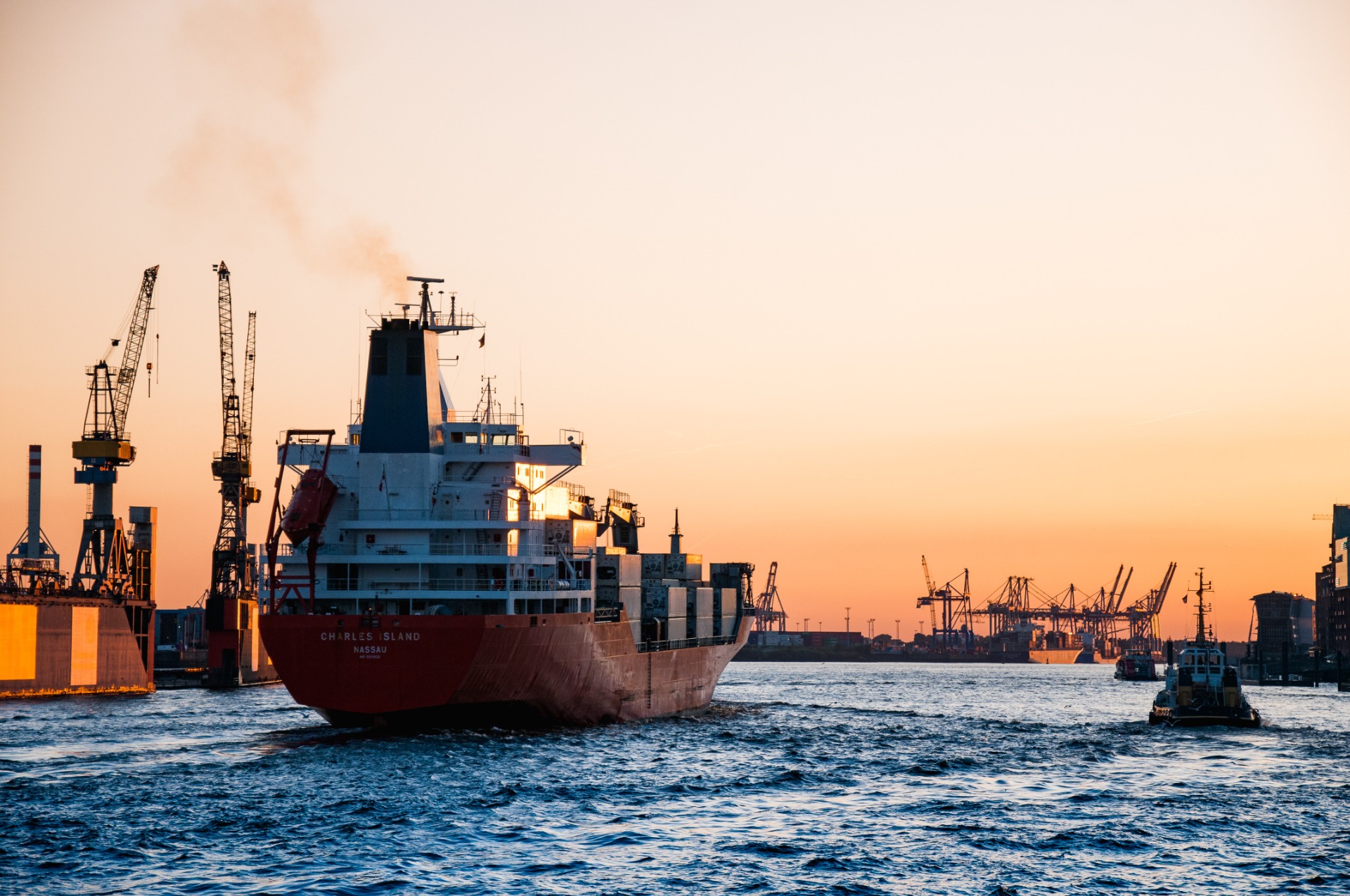
In this post I will describe how to serve your Django and React routes from the same port. Before we get started, your project structure should look something like this:
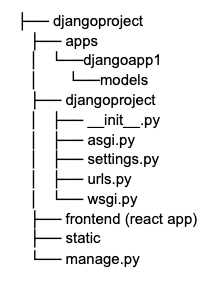
1. In djangoproject/settings .py add the following lines of code.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# in settings.py | |
import os # 1. Add this import | |
TEMPLATES = [ | |
{ | |
'BACKEND': 'django.template.backends.django.DjangoTemplates', | |
# 2. Add the root folder of frontend to serve front and back end at port 8000. | |
'DIRS': [os.path.join(BASE_DIR, 'frontend')], | |
'APP_DIRS': True, | |
'OPTIONS': { | |
'context_processors': [ | |
'django.template.context_processors.debug', | |
'django.template.context_processors.request', | |
'django.contrib.auth.context_processors.auth', | |
'django.contrib.messages.context_processors.messages', | |
], | |
}, | |
}, | |
] | |
STATICFILES_DIRS = ( | |
# 3. Add the build directory from the frontend directory to serve front and back end at port 8000. | |
os.path.join(BASE_DIR, 'frontend', "build", "static"), | |
) |
2. In djangoapp1/views add a view to render the frontend index.html file from the build directory.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# in views.py | |
def index(request): | |
# Add a view that renders the frontend build directory to serve front and back end at port 8000. | |
return render(request, "build/index.html") |
3. In djangoproject/urls.py add the path to the view we just created.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
# urls.py | |
from backend_apps.food_app.views import index | |
urlpatterns = [ | |
path('admin/', admin.site.urls), | |
# Route “host:8000/” to the view that renders the frontend build to serve front and back end at port 8000. | |
path("", index, name="index"), | |
] |
Now if you:
- cd djangoproject/frontend
- install your node packages: npm install
- create your build directory: npm run build
- start your frontend server: npm start
- cd ..
start your virtual env: source env/bin/activate (or however you start your virtual env)
start your backend server: python manage.py runserver
You should be able to see your react app at localhost: 8000/!
Feel free to leave me a comment to let me know what you thought of this article. Thank you!